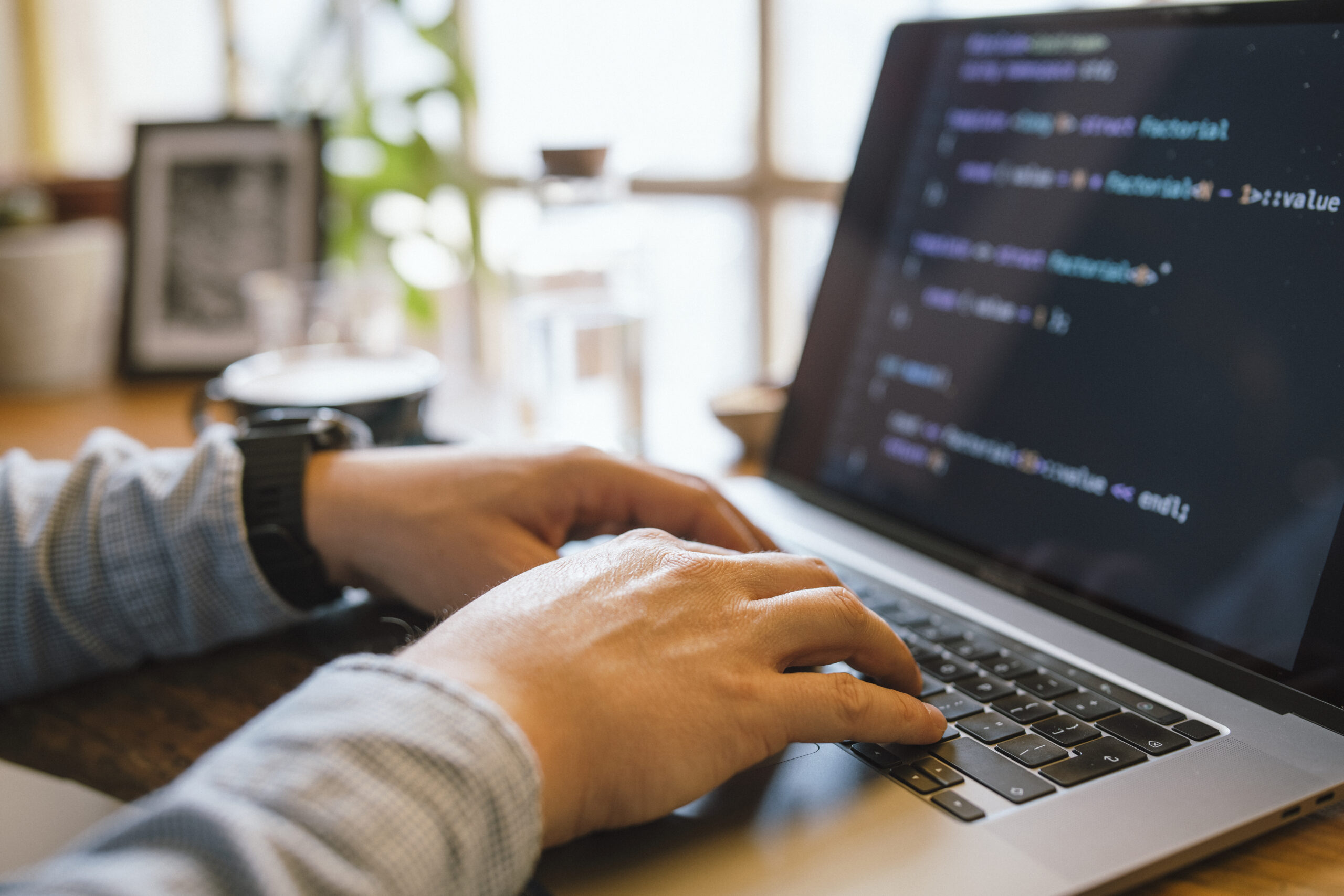
Debugging is The most essential — however usually neglected — competencies in a developer’s toolkit. It isn't just about correcting damaged code; it’s about being familiar with how and why matters go wrong, and Studying to Believe methodically to unravel challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Listed below are numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Learn Your Applications
Among the list of fastest techniques developers can elevate their debugging skills is by mastering the resources they use every day. Though producing code is one particular Portion of growth, recognizing the way to interact with it successfully during execution is Similarly critical. Fashionable enhancement environments appear equipped with powerful debugging abilities — but a lot of builders only scratch the area of what these equipment can do.
Get, as an example, an Integrated Enhancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, phase by means of code line by line, as well as modify code around the fly. When employed accurately, they Allow you to observe precisely how your code behaves during execution, and that is invaluable for monitoring down elusive bugs.
Browser developer equipment, like Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, check community requests, see real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Handle about running processes and memory management. Mastering these tools might have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation Manage techniques like Git to be aware of code history, discover the exact second bugs have been released, and isolate problematic changes.
In the end, mastering your resources implies heading over and above default options and shortcuts — it’s about developing an intimate knowledge of your improvement atmosphere in order that when troubles come up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most significant — and infrequently forgotten — techniques in powerful debugging is reproducing the trouble. Just before leaping in to the code or creating guesses, developers have to have to make a steady atmosphere or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of probability, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise situations below which the bug takes place.
As soon as you’ve gathered ample information, try and recreate the issue in your local setting. This may indicate inputting the same knowledge, simulating identical user interactions, or mimicking method states. If The problem seems intermittently, contemplate producing automated exams that replicate the sting instances or point out transitions concerned. These assessments not only aid expose the condition but additionally avert regressions Later on.
From time to time, The difficulty could be natural environment-specific — it might come about only on sure working devices, browsers, or under specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires patience, observation, and also a methodical solution. But when you can persistently recreate the bug, you happen to be by now midway to correcting it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, take a look at probable fixes properly, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders should learn to take care of error messages as direct communications from the procedure. They generally let you know just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the information very carefully and in whole. Several developers, specially when below time tension, look at the primary line and right away start generating assumptions. But deeper from the error stack or logs may perhaps lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — browse and recognize them initial.
Split the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line variety? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also handy to know the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Studying to acknowledge these can drastically hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context through which the error occurred. Check out linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a additional economical and confident developer.
Use Logging Properly
Logging is The most highly effective instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with realizing what to log and at what degree. Typical logging ranges include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic data for the duration of growth, Information for common events (like successful start out-ups), Alert for prospective troubles that don’t break the application, Mistake for true issues, and Lethal if the program can’t carry on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target important situations, point out alterations, input/output values, and important selection details with your code.
Format your log messages Plainly and constantly. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs Allow you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-imagined-out logging solution, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the General maintainability and dependability within your code.
Think Like a Detective
Debugging is not simply a complex endeavor—it's a type of investigation. To properly determine and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This frame of mind helps break down sophisticated troubles into workable sections and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much suitable info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s going on.
Next, sort hypotheses. Check with on your own: What may be triggering this conduct? Have any adjustments lately been produced on the codebase? Has this situation occurred before less than very similar conditions? The aim is always to narrow down alternatives and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the trouble in a managed setting. In the event you suspect a selected purpose or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the results guide you closer to the reality.
Pay out shut notice to modest details. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely being familiar with it. Short term fixes may perhaps conceal the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for potential difficulties and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed issues in sophisticated devices.
Generate Tests
Creating assessments is among the simplest tips on how to enhance your debugging techniques and overall improvement efficiency. Exams not merely enable capture bugs early but will also function a security Web that offers you self-assurance when generating alterations on your codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue happens.
Begin with device exams, which target specific features or modules. These modest, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Functioning as anticipated. Whenever a test fails, you immediately know where by to glimpse, appreciably cutting down enough time invested debugging. Unit checks are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These support make sure that various aspects of your application work alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated units with a number of parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To check a attribute properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to raised code structure and less bugs.
When debugging an issue, producing a failing test that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on fixing the bug and enjoy your test pass when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, minimize stress, and sometimes see The problem from a new viewpoint.
When you're way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso split, and even switching to a special job for ten–quarter-hour can refresh your target. Several developers report discovering the root of a challenge once they've taken time for you to disconnect, letting their subconscious get the job done while in the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
If you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It could come to feel counterintuitive, especially underneath tight deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a sensible technique. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, every one can teach you some thing worthwhile when you take the time to reflect and examine what went Erroneous.
Get started by inquiring yourself a couple of important queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code opinions, or logging? The responses generally expose blind places in the workflow or being familiar with and help you build much better coding patterns going ahead.
Documenting bugs can even be a fantastic practice. Hold a developer journal or keep a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be especially impressive. No matter if it’s by way of a Slack message, a brief produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, read more viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your advancement journey. After all, several of the very best builders aren't those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies requires time, exercise, and tolerance — however the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep inside a mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.